Carbon.js
14/02/2023
This is a Carbon like image generator from code.
I decided to create a Node.js library available for free on NPM, taking the idea of the Carbon website, allowing to transform code into JPEG image. Most (if not all) of the other projects used :
- Either APIs hosted on free services and more maintained to go scrape the Carbon website.
- Or directly used a very poorly optimized technique, i.e. directly scrapping the code via puppeteer on the website.
These techniques, in addition to not being very optimized, take a lot of time for the simple generation of an image.
So I wanted to create a library that would render a similar image, but faster and in a more optimized way.
Technologies used
I decided to used TypeScript for this project, to learn how I can create an NPM library with TypeScript.
For the technical reasons stated in the previous point, I also decided to use only “small libraries”:
- The first one has been designed to tokenize the code entered by the user. I chose prismjs, for the simple reason that hljs was a bit of a hellish library in terms of scale, and didn’t detect correctly the programming language used. Therefore, I decided to remove this feature, and to switch to prismjs.
- For the Image Generation, I used the most common library in Node.js which is the Canvas library.
And of course, a lot of math! You can choose your preferred width, create new themes (feature under development), and render your images in PNG, JPEG, and PDF!
Example
The input code :
const pluckDeep = (key) => (obj) =>
key.split(".").reduce((accum, key) => accum[key], obj);
const compose =
(...fns) =>
(res) =>
fns.reduce((accum, next) => next(accum), res);
const unfold = (f, seed) => {
const go = (f, seed, acc) => {
const res = f(seed);
return res ? go(f, res[1], acc.concat([res[0]])) : acc;
};
return go(f, seed, []);
};
The output image :
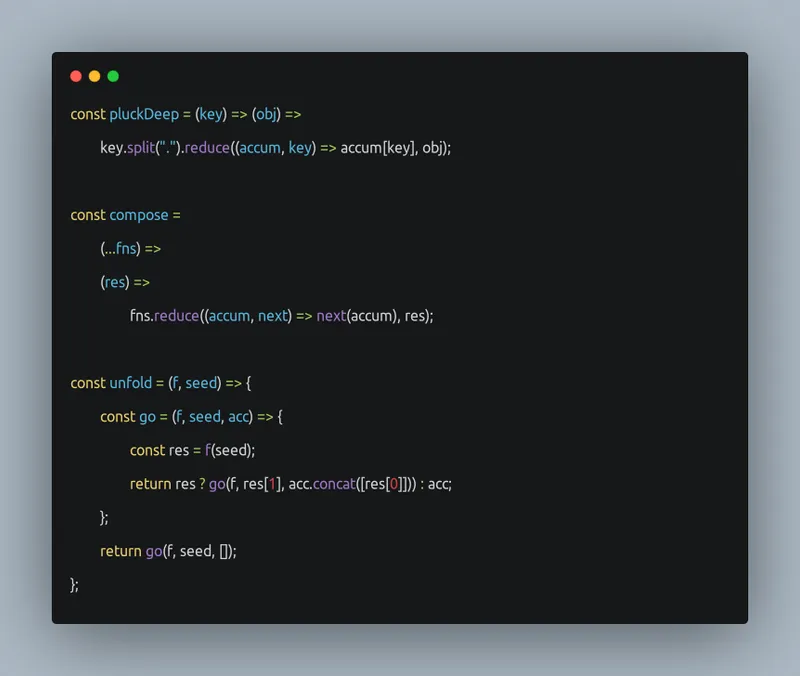